#include "StuDraw3DEvent.h"
#include "TVirtualPad.h"
#include "TColor.h"
#include "StEventHelper.h"
#include "StEvent.h"
#include "StTrack.h"
#include "StHit.h"
#include "StTpcHit.h"
#include "StTrackNode.h"
#include "StTrackGeometry.h"
#include "StTpcHitCollection.h"
#include "StMeasuredPoint.h"
#include "TMath.h"
#include "StTrackDetectorInfo.h"
ClassImp(StuDraw3DEvent)
> 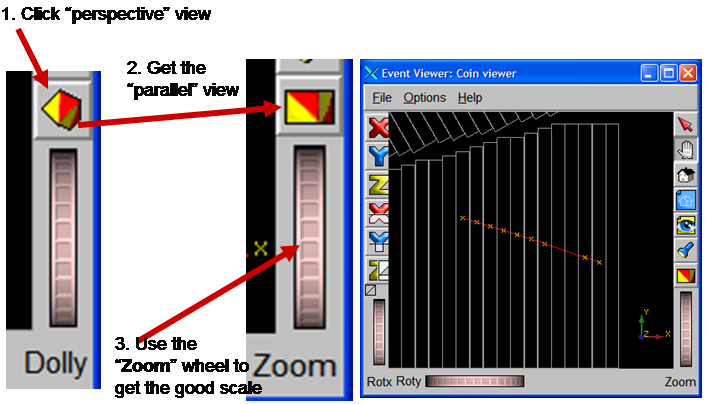
StuDraw3DEvent::StuDraw3DEvent( const char *detectorName,TVirtualPad *pad):
StDraw3D(detectorName,pad)
{
if (!gEventDisplay) gEventDisplay = this;
}
StuDraw3DEvent::~StuDraw3DEvent()
{
if (gEventDisplay == this) gEventDisplay = 0;
}
TObject *StuDraw3DEvent::Track(const StTrack &track, Color_t col,Style_t sty,Size_t siz)
{
StTrackPoints trPnt(&track);
TObject *l = Line(trPnt.Size(),trPnt.GetXYZ(0),col,sty,siz);
SetModel((TObject*)&track);
return l;
}
TObject *StuDraw3DEvent::Track(const StTrack &track, EDraw3DStyle sty)
{
const StDraw3DStyle &style = Style(sty);
return Track(track, style.Col(),style.Sty(),style.Siz() );
}
TObject *StuDraw3DEvent::Hit(const StMeasuredPoint &hit
, Color_t col, Style_t sty, Size_t siz)
{
const StThreeVectorF& position = hit.position();
TObject *p = Point(position.x(),position.y(),position.z(),col,sty,siz);
SetModel((TObject*)&hit);
return p;
}
TObject *StuDraw3DEvent::Hit(const StMeasuredPoint &hit, EDraw3DStyle sty)
{
const StDraw3DStyle &style = Style(sty);
return Hit(hit, style.Col(),style.Sty(),style.Siz() );
}
void StuDraw3DEvent::Hits(const StTrack &track, EDraw3DStyle sty)
{
const StDraw3DStyle &style = Style(sty);
Hits(track, style.Col(),style.Sty(),style.Siz());
}
void StuDraw3DEvent::Hits(const StTrack &track)
{
if (track.flag() > 0 && track.detectorInfo()&& !track.bad() ) {
const Int_t lightness = 50;
const Int_t saturation = 100;
Int_t hue = 0;
Style_t sty = Style(kUsedHit).Sty();
Size_t siz = Style(kUsedHit).Siz();
double pt = track.geometry()->momentum().perp();
hue = Int_t(256.*(1.-pt/1.5));
if (pt > 1.5 ) hue = 0;
Int_t r,g,b;
TColor::HLS2RGB(hue, lightness, saturation, r, g, b);
float factor = 1./TMath::Sqrt(1.*r*r+1.*g*g+1.*b*b);
Color_t trackColor = TColor::GetColor(r*factor,g*factor,b*factor);
Hits(track, trackColor,sty,siz);
}
}
void StuDraw3DEvent::Hits(const StTrack &track
, Color_t col
, Style_t sty
, Size_t siz )
{
std::vector<float> hitPoints;
const StPtrVecHit& trackHits = track.detectorInfo()->hits(kTpcId);
for (unsigned int m=0; m<trackHits.size(); m++) {
StHit *hit = trackHits[m];
hitPoints.push_back( hit->position().x());
hitPoints.push_back( hit->position().y());
hitPoints.push_back( hit->position().z());
}
std::vector<float>::iterator xyz = hitPoints.begin();
Points(hitPoints.size()/3,&*xyz,col,sty,siz);
}
TObject *StuDraw3DEvent::Vertex(const StMeasuredPoint &vertex
, Color_t col, Style_t sty, Size_t siz)
{
return Hit(vertex,col,sty,siz);
}
TObject *StuDraw3DEvent::Vertex(const StMeasuredPoint &vtx, EDraw3DStyle sty)
{
const StDraw3DStyle &style = Style(sty);
return Vertex(vtx, style.Col(),style.Sty(),style.Siz() );
}
TObject *StuDraw3DEvent::TrackInOut(const StTrack &track, Bool_t in
, Color_t col, Style_t sty, Size_t siz)
{
StInnOutPoints trInOut(&track,in);
return Points(trInOut.Size(),trInOut.GetXYZ(0),col,sty,siz);
}
TObject *StuDraw3DEvent::TrackInOut(const StTrack &track, EDraw3DStyle sty,Bool_t in)
{
const StDraw3DStyle &style = Style(sty);
return TrackInOut(track, in, style.Col(),style.Sty(),style.Siz() );
}
void StuDraw3DEvent::Tracks(const StEvent* event, StTrackType type)
{
Hits(event,kTracksOnly,type);
}
void StuDraw3DEvent::Tracks(const StSPtrVecTrackNode &theNodes
, StTrackType type)
{
const Int_t lightness = 50;
const Int_t saturation = 100;
Int_t hue = 0;
StTrack *track;
StThreeVectorD p;
unsigned int i;
for (i=0; i<theNodes.size(); i++) {
track = theNodes[i]->track(type);
if (track && track->flag() > 0
)
{
double pt = track->geometry()->momentum().perp();
hue = Int_t(256.*(1.-pt/1.5));
if (pt > 1.5 ) hue = 0;
Int_t r,g,b;
TColor::HLS2RGB(hue, lightness, saturation, r, g, b);
float factor = 1./TMath::Sqrt(1.*r*r+1.*g*g+1.*b*b);
Color_t trackColor = TColor::GetColor(r*factor,g*factor,b*factor);
Track(*track,trackColor);
}
}
}
void StuDraw3DEvent::Hits(const StEvent *event,EStuDraw3DEvent trackHitsOnly, StTrackType type)
{
if (!event) return;
const StTpcHitCollection* hits = event->tpcHitCollection();
if (!hits) return;
unsigned int m, n, h;
if (trackHitsOnly != kUnusedHitsOnly) {
const Int_t lightness = 50;
const Int_t saturation = 100;
Int_t hue = 0;
Style_t sty = Style(kUsedHit).Sty();
Size_t siz = Style(kUsedHit).Siz();
Style_t styPnt = Style(kTrackBegin).Sty();
Size_t sizPnt = Style(kTrackBegin).Siz();
const StSPtrVecTrackNode& theNodes = event->trackNodes();
for (unsigned int i=0; i<theNodes.size(); i++) {
StTrack *track = theNodes[i]->track(type);
if (track && track->flag() > 0
&& track->detectorInfo()
&& !track->bad()
)
{
double pt = track->geometry()->momentum().perp();
hue = Int_t(256.*(1.-pt/1.5));
if (pt > 1.5 ) hue = 0;
Int_t r,g,b;
TColor::HLS2RGB(hue, lightness, saturation, r, g, b);
float factor = 1./TMath::Sqrt(1.*r*r+1.*g*g+1.*b*b);
Color_t trackColor = TColor::GetColor(r*factor,g*factor,b*factor);
if ( trackHitsOnly != kUsedHits) {
Track(*track,trackColor);
TrackInOut(*track, true, trackColor, styPnt, sizPnt);
TrackInOut(*track, false, trackColor, styPnt, sizPnt);
}
if ( trackHitsOnly != kTracksOnly) {
Hits(*track,trackColor,sty,siz);
if (trackHitsOnly == kUsedHits) SetModel(track);
}
}
}
} else {
const StTpcHit *hit;
std::vector<float> hitPoints;
for (n=0; n<hits->numberOfSectors(); n++) {
for (m=0; m<hits->sector(n)->numberOfPadrows(); m++) {
for (h=0; h<hits->sector(n)->padrow(m)->hits().size(); h++) {
hit = hits->sector(n)->padrow(m)->hits()[h];
hitPoints.push_back( hit->position().x());
hitPoints.push_back( hit->position().y());
hitPoints.push_back( hit->position().z());
}
}
}
std::vector<float>::iterator xyz = hitPoints.begin();
Points(hitPoints.size()/3,&*xyz,kUnusedHit);
SetComment("Unused TPC hits");
}
}
StuDraw3DEvent *StuDraw3DEvent::Display(){ return gEventDisplay;}
StuDraw3DEvent *gEventDisplay = new StuDraw3DEvent();
ROOT page - Class index - Class Hierarchy - Top of the page
This page has been automatically generated. If you have any comments or suggestions about the page layout send a mail to ROOT support, or contact the developers with any questions or problems regarding ROOT.